Reverse Iterator C++ Set
Here we will easily learn, how to reverse a vector in c++.
Reverse iterator c++ set. Consequently, we have reverse. The function does not take any parameter. Many containers implement the bidirectional iterator such as list, set, multiset, map, multimap.
If you have an existing map object that is not created using the reverseOrder method, you can use the below given approaches to iterate the map in reverse order. Declare the reverse iterator on this set. The above given approach works at the TreeMap object creation time.
C++ set is an inbuilt associative container that contains unique objects because the value of the elements identifies the container. Rbegin points to the element preceding the one that would be pointed to by member end. So it seems easy to reverse the elements of a vector by the help of header file algorithm.
An iterator can be incremented or decremented but it cannot modify the content of deque. C++ provides two non-const iterators that move in both the directions are iterator and reverse iterator. Being templates, they can be used to store arbitrary elements, such as integers or custom classes.The following containers are defined in the current revision of the C++ standard:.
If you can use a string than you can use a reverse iterator. Here we will use the rbegin() and rend() function to get the begin and end of the. Returns a reverse iterator pointing to the theoretical element right before the first element in the multiset container.
++iter reads the next value from the stream and returns its position. Get code examples like "iterate through unordered_map c++ in reverse order" instantly right from your google search results with the Grepper Chrome Extension. Increasing them moves them towards the beginning of the container.
When compiling in default (standard) mode, the compiler has access to the complete library specified by the C++ standard. Returns an iterator pointing to the first element in the set container which is not considered to go before value. The end() function returns an iterator just past the end of the vector.
In this post, we will see how to reverse a vector in C++. Using the C++ iterator, operator+ to add an offset to an iterator and returns a move_iterator or a reverse_iterator addressing the inserted element at the new offset position in C++ programming. Parameters none Return Value A reverse iterator to the reverse end of the sequence container.
So if the set is like S = 10, 15, 26, 30, 35, 40, 48, 87, 98, then the output will be:. C++ specification of `std::reverse_iterator` makes creating a `std::string_view` from another `std::string_view`'s reverse iterators impossible. As we saw when working on dynamic bitsets, it can be useful to traverse a collection backwards, from its last element to its first one.
Used by an iterator-- obsolete __iterator_category:. Reverse_iterator::reverse_iterator(iterator) constructs a reverse iterator from an iterator. A container that organizes a set of sequences:.
We can also use reverse iterators to construct a new vector using. For a vector or a list, a reverse iterator generates the last element first, and the first element last. Now we want to iterate over this list in reverse order( from end to start ) i.e.
Moreover, reverse() is a predefined function in the header file algorithm. Searches the set container for value and returns an iterator to it if found, else returns an iterator to set::end. // Creating a reverse iterator pointing to end of set i.e.
If the set object is const-qualified, the function. Similarly, we call the previous method of the ListIterator when the next method of custom iterator is called. End() – Returns an iterator past the last element of the set.
But we cannot decrement forward iterators, input iterators, and output. Where, rbegin() stands for reverse beginning. Of is that this hello hi We don’t want to change the order in existing list, just want to iterate in reverse.
This is a quick tutorial on how to use reverse iterator in C++. All containers in C++ do not support all iterators. Iter = value writes value to ostream, followed by delim if set.
Compiled as C++ Code (/TP) Other info:. This is equivalent to ostream << value << delim. Reverse_iterator<Iterator>::base() is required to return Iterator.
Make_reverse_iterator is a convenience function template that constructs a std::reverse_iterator for the given iterator i (which must be a LegacyBidirectionalIterator) with the type deduced from the type of the argument. To iterate backwards use rbegin() and rend() as the iterators for the end of the collection, and the start of the collection respectively. Set, map, multiset, multimap.
A constant iterator allows you to read but not modify the contents of the vector which is useful to enforce const correctness:. This is a guest post by Carlos Buchart.Carlos is one of the main C++ developers at the Motion Capture Division of STT Systems, author of HeaderFiles (in Spanish) and a Fluent C++ follower. We must initialize an iterator which will be responsible for traversing the set and accessing each element.
Suppose we have a set, then we have to traverse the set in reverse direction. 98 87 48 40 35 30 26 15 10. If you're really stuck with C++11, then we'll have to add the trailing return type:.
#include <vector> iterator end();. To iterate backwards use rbegin() and rend() as the iterators for the end of the collection, and the start of the. In computing, associative containers refer to a group of class templates in the standard library of the C++ programming language that implement ordered associative arrays.
Rbegin std::set<std::string>::reverse_iterator revIt. Set project to be compiled as C++. Print set in reverse order.
In other words, when provided with a bidirectional iterator, std::reverse_iterator produces a new iterator that moves from the end to the beginning of the sequence defined by the underlying bidirectional iterator. In this tutorial, we are going to learn how to decrement an iterator in C++. See the example for rbegin for an example of how to declare and use reverse_iterator.
It would be nice to be able to use C++11 range for loops to iterate. For example, an array container might offer a forwards iterator that walks through the array in forward order, and a reverse iterator that walks through the array in reverse order. Returns a reverse iterator pointing to the last element in the multiset container.
So we internally call the hasPrevious method of the ListIterator when the hasNext method of custom iterator is called. A type const_reverse_iterator cannot modify the value of an element and is use to iterate through the hash_set in reverse. Reverse iterators are those which iterate from backwards and move towards the beginning of the deque.
Since C++11 the cbegin() and cend() methods allow you to obtain a constant iterator for a vector, even if the vector is non-const. See the example for rend for an example of how to declare and use the const_reverse_iterator. Returns a constant iterator pointing to the first element in the container.
A type reverse_iterator is used to iterate through the set in reverse. For this to work properly, we need to change the return type of begin() and end() to auto, so that we get const_reverse_iterator back from them. The range between set::rbegin and set::rend contains all the elements of the container (in reverse order).
To traverse in reverse order, we can use the reverse_iterator. Program to demonstrate the set::rbegin() method:. To traverse a Set in reverse order, a reverse_iterator can be declared on it and it can be used to traverse the set from the last element to the first element with the help of rbegin() and rend() functions.
List reverse iterator declaration:. In this example we define a set of integer and with the help of reverse iterator print the reverse order of our set. Using the C++ reverse_iterator::base() to recover the underlying iterator from its reverse_iterator in C++ programming.
So that it points to the last element of set and then we will keep on access and increment the iterator to next till set::rend() is reached i.e. If we want to iterate backwards through a list or vector we can use a reverse_iterator.A reverse iterator is made from a bidirectional, or random access iterator which it keeps as a member which can be accessed through base(). Note that before you can access the last element of the vector using an iterator that you get from a call to end(), you'll have to decrement the iterator first.
We can only decrement random access iterators and bidirectional iterators. Traverse the set from the last element to the first element with the help of rbegin. See the very bottom of the post.
Do you have to work with a char * or can you use a string?If you have to use a char * then you need to know the legnth of the string and then you can use a for loop to loop from the back of the string to 0. Std::reverse_iterator is an iterator adaptor that reverses the direction of a given iterator. Take the resulting iterator, construct a reverse iterator from it, and assign it to the reverse.
For a set it generates the largest element first, and the smallest element last. Determines the category to which an iterator belongs--obsolete __reverse. List::rbegin() and list::rend() functions.
The function returns a reverse iterator pointing to the element right before the first element in the set container. C++ Bidirectional iterator has the same features like the forward iterator, with the only difference is that the bidirectional iterator can also. Compiled as C++ Code (/TP) Other info:.
Istream_iterator<T>(istream) creates an istream iterator for an input stream (istream) and reads the first value. These are used to traverse through the elements of the containers. Begin() – Returns an iterator to the first element of the set.
Reverse iterators iterate backwards:. For a reverse iterator r constructed from an iterator i, the relationship & * r. Istream_iterator<T>() creates an end-of-stream iterator.
They store unique elements in a particular order. Simplest solution is to use std::reverse function defined in the header. For my own convenience, I'll work with C++17 from here.
Put altogether, the line it = rev_itr(v.erase(it.base())) says:. Auto begin()-> decltype(m_container.begin()), for example. A container may provide different kinds of iterators.
Firstly, we will store the numbers in a vector and we will create an iterator for it. This whole thing was nonsense. This example will show you how to iterate to a vector of struct that consists of multiple members.
*iter returns the value that was read before. Returns a reverse iterator pointing to the theoretical element right before the first element in the set container (which is considered its reverse end). Strictly speaking, reverse iterators do not constitute a new category of iterator, but an adaptation of another iterator type.
The value of the elements in a set cannot be modified once they are put into the container, but other operations such as removal and insertion on the container can take place. Traversing an Unordered Set. Take the reverse iterator it, have v erase the element pointed by its regular iterator;.
A custom Iterator must implement three methods hasNext, next and remove.Basically, we have wrapped the ListIterator into a custom Iterator. Modify is a char * not a string therefore it does not have rbegin() or rend() member function. Set::rbegin() is a built-in function in C++ STL which returns a reverse iterator pointing to the last element in the container.
When Iterator is vector<int>::iterator, if that happens to be int *, then --ri.base() must fail to compile (because int * is not of class type). If the set object is const-qualified, the. The C++ Standard Library.
Project -> your_project_name Properties -> Configuration Properties -> C/C++ -> Advanced -> Compiled As:. In this post, we will discuss various methods to iterate over Set in Java. If we want to iterate backwards through a list or vector we can use a reverse_iterator.A reverse iterator is made from a bidirectional, or random access iterator which it keeps as a member which can be accessed through base().
A type that provides a bidirectional iterator that can read or modify an element in a reversed set. Parameters none Return Value A reverse iterator to the reverse beginning of the sequence container. C++ Deque rbegin() function returns a reverse iterator pointing to the last element of the container.
To iterate a list in reverse order in C++ STL, we need an reverse_iterator that should be initialized with the last element of the list, as a reverse order and we need to check it till the end of the list. Now to iterate a set in reverse direction, we need to create an reverse_iterator and initialise it with set::rbegin(). Using the descendingKeySet method of the TreeMap class.
This function internally uses std::iter_swap for swapping the elements from both ends of the given range. Now let’s see how to do this using different techniques, Iterate over the list in reverse using while loop. Unordered sets support two built-in functions that return an iterator.
The function returns a reverse iterator pointing to the last element in the container. Project -> your_project_name Properties -> Configuration Properties -> C/C++ -> Advanced -> Compiled As:. We can use iterator() that returns an iterator to iterate over a set as shown below:.
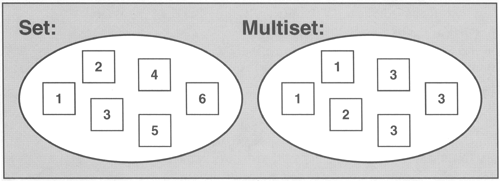
Std Set C Ccplusplus Com
Q Tbn 3aand9gcs C5o Strnt9cihjlx4qdgazg Bhr3ikjqz4 S4gokol1uwpin Usqp Cau

Why Does A Push Back On An Std List Change A Reverse Iterator Initialized With Rbegin Stack Overflow
Reverse Iterator C++ Set のギャラリー

Stl Container For C Knowledge Sharing Simple Application Of Set Container And Map Container Programmer Sought

Apostila Programacao Orientada A Objeto Com C Stl Referencia Programacao Orientada Docsity
C Set Rbegin Function Alphacodingskills
Iterators Json For Modern C

Bidirectional Iterators In C Geeksforgeeks
C Stl Containers Summary Vector List Deque Queue Stack Set Map Programmer Sought
2

The Standard Template Library Ppt Download
Solved Create A C Class Similar To Stl Maps Here Are T Chegg Com

Python Program To Iterate Over The List In Reverse Order Codevscolor
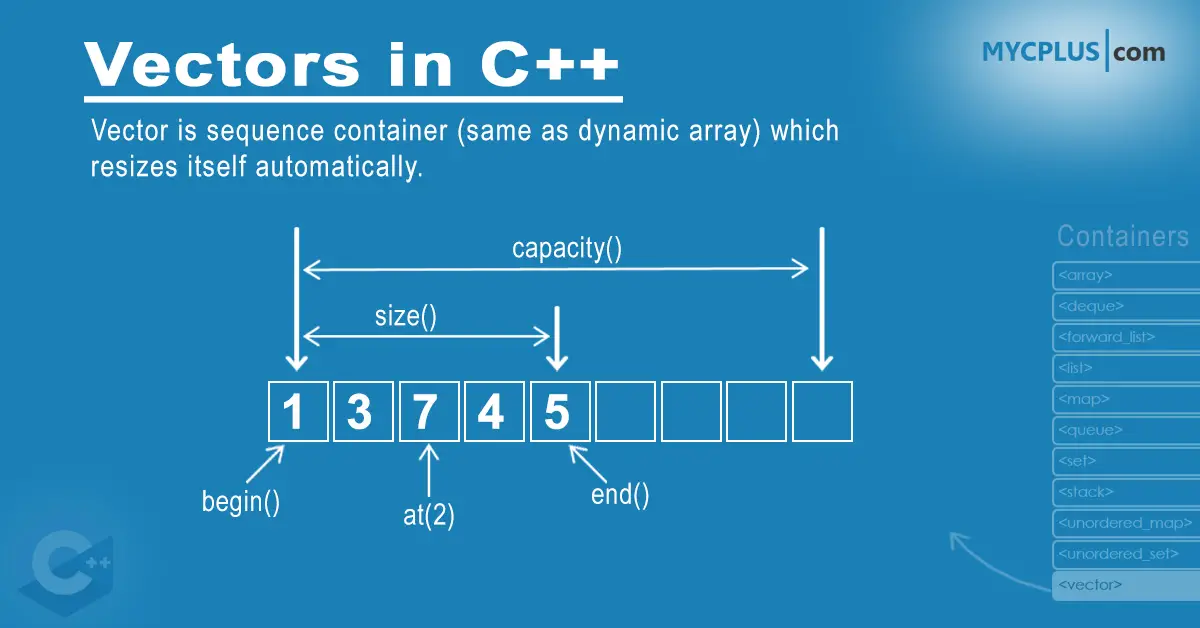
C Vectors Std Vector Containers Library Mycplus

Stl Container For C Knowledge Sharing Simple Application Of Set Container And Map Container Programmer Sought
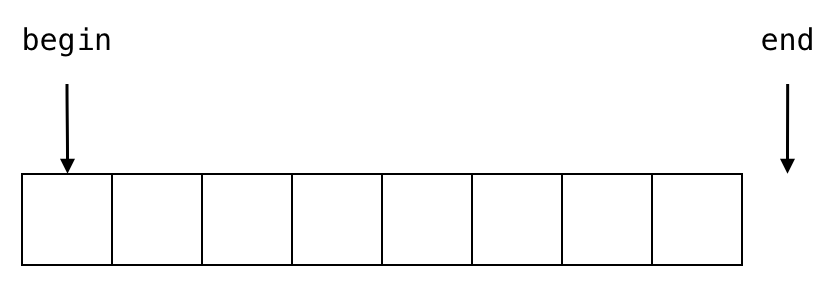
Iterators Data Structures
C Iterator Programmer Sought
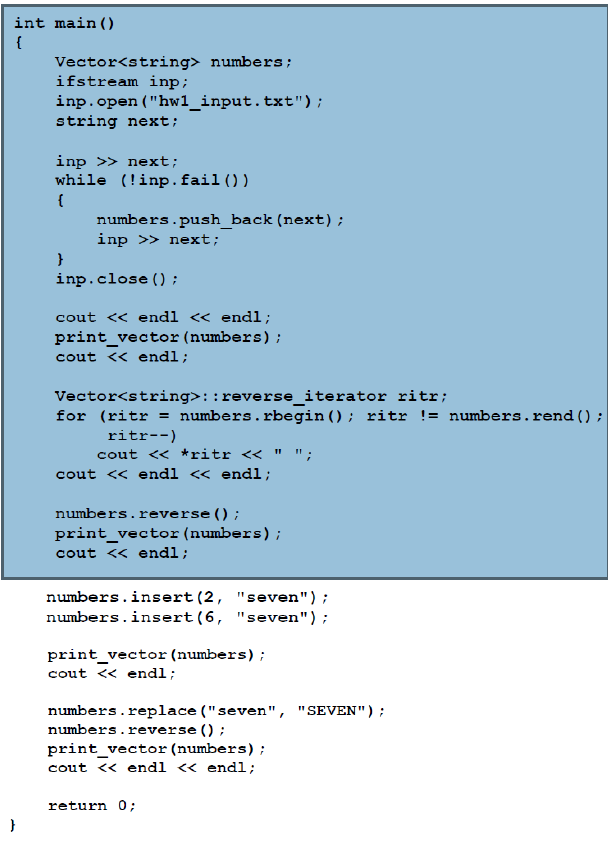
Solved Please Use C Please Help Thank You So Much Ad Chegg Com
Reverse A Number In C Program Code C Programming Tutorial For Beginners
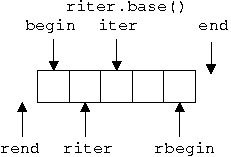
Stl Iterators
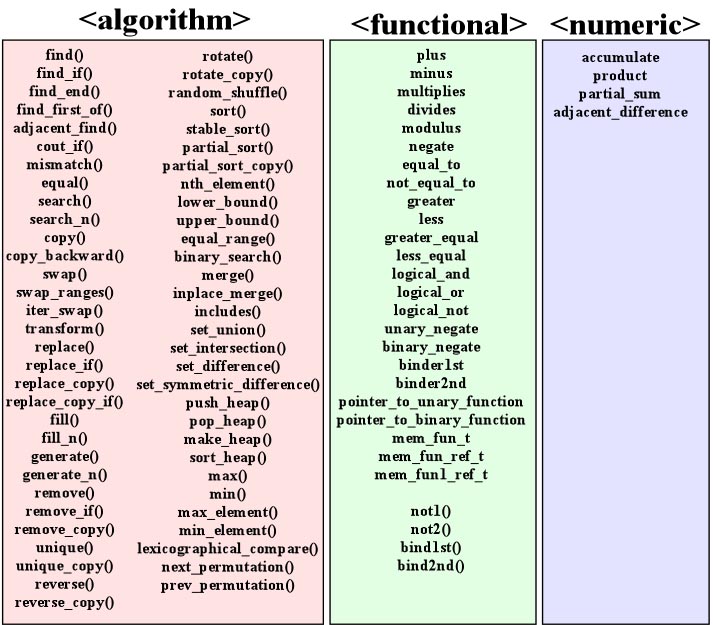
Cs 307
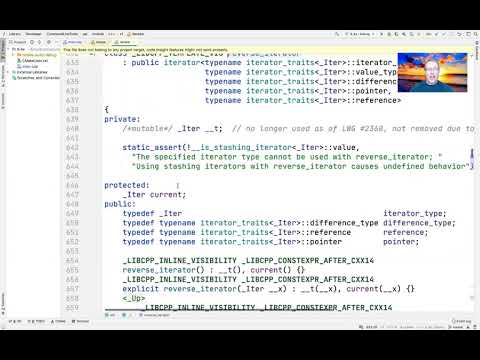
C Stl Iterator Adapters Part 2 Youtube

The C Standard Template Library Stl Programming On Iterators Algorithm And Containers Tutorials With C Program Examples
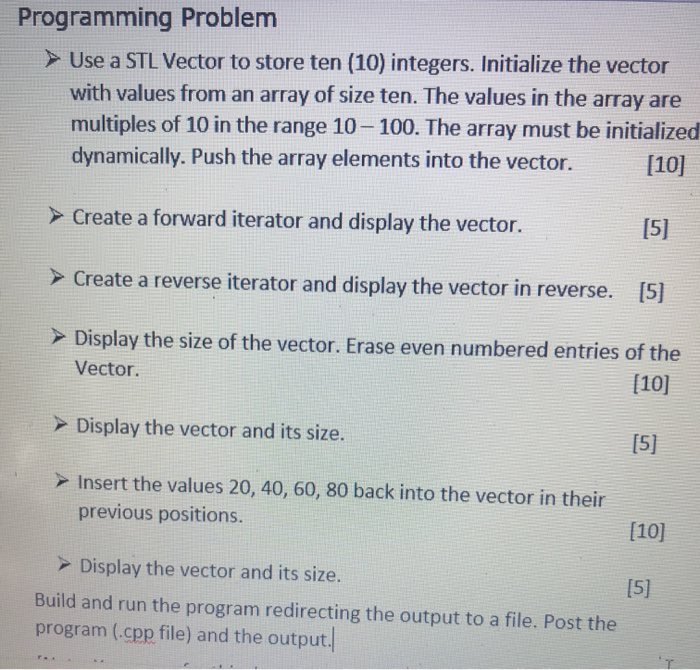
Solved Programming Problem Use A Stl Vector To Store Ten Chegg Com
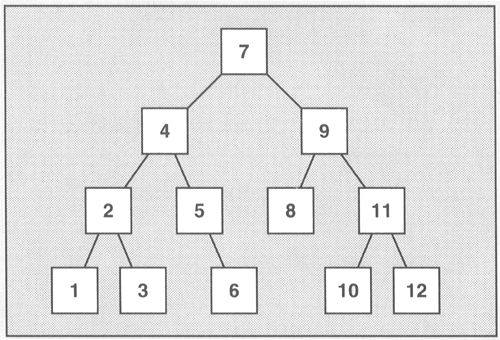
Std Set Example C Ccplusplus Com

Libstdc Std List Class Reference
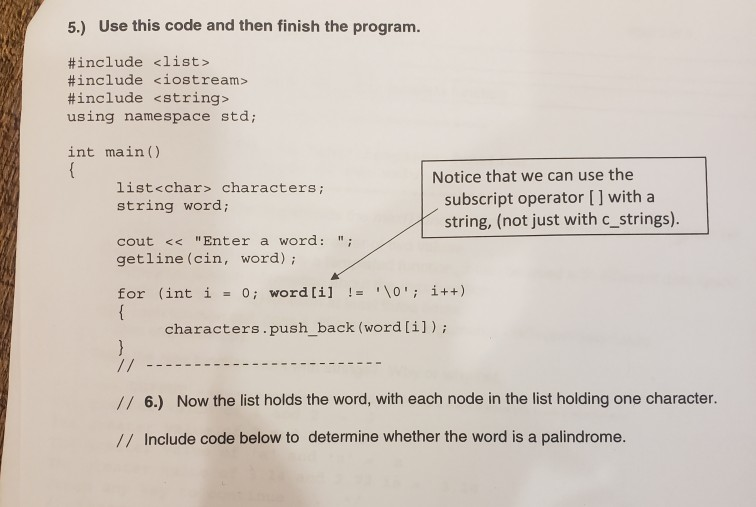
Solved Hw 10d Palindrome Using List And Iterator And Chegg Com
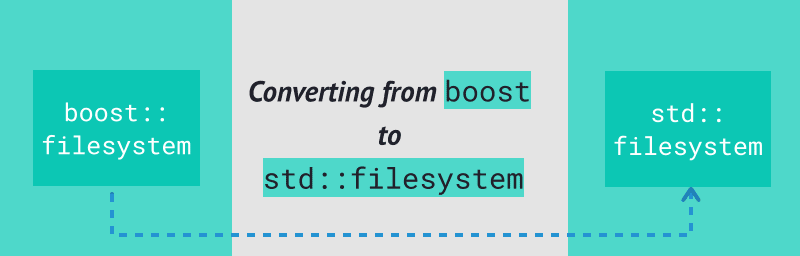
Bartek S Coding Blog Converting From Boost To Std Filesystem
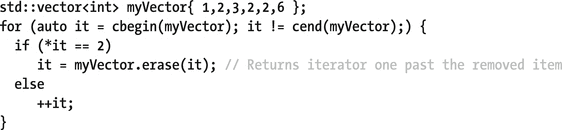
Containers Springerlink

Find All Permutations Of A String In C Using Backtracking And Stl Techie Delight

Iterator Pattern Wikipedia

The Difference Between Std Copy Backward And Std Copy With Reverse Iterators Fluent C
1
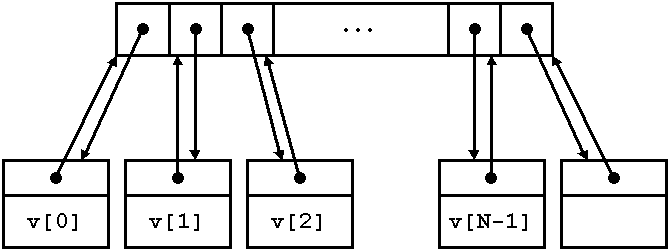
Non Standard Containers
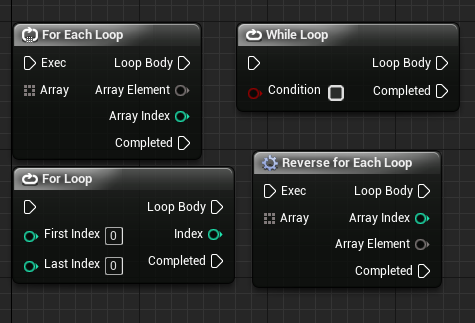
How To Use Loops And Arrays Unreal Engine 4 Couch Learn
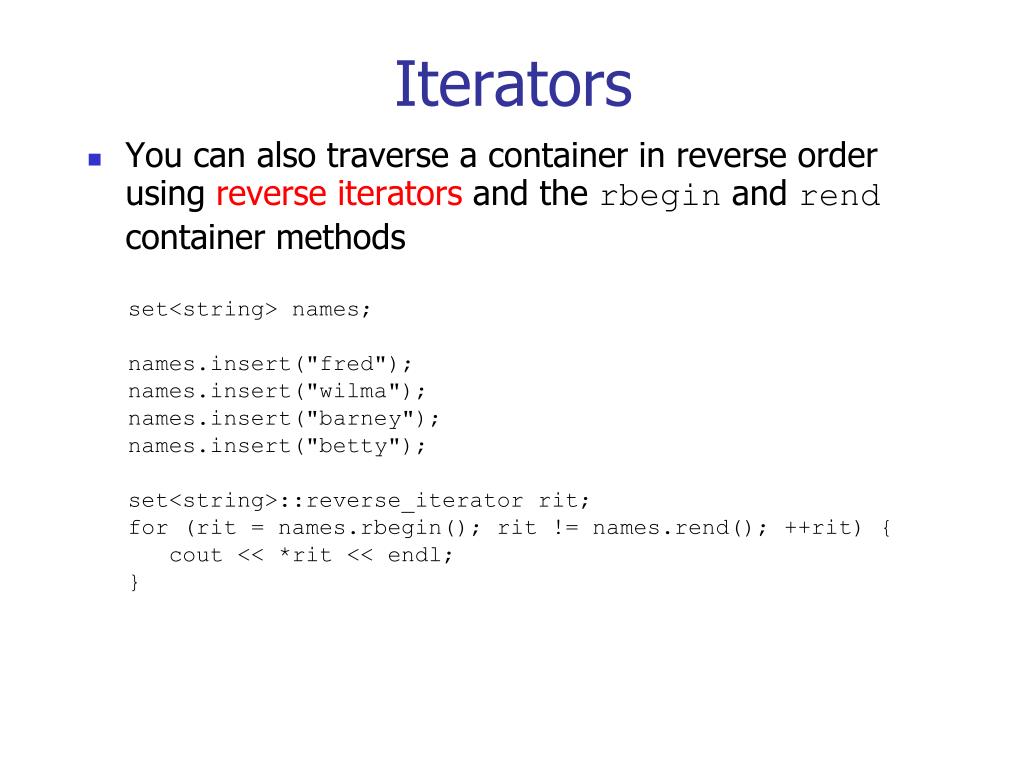
Ppt Standard Template Library Stl Powerpoint Presentation Free Download Id
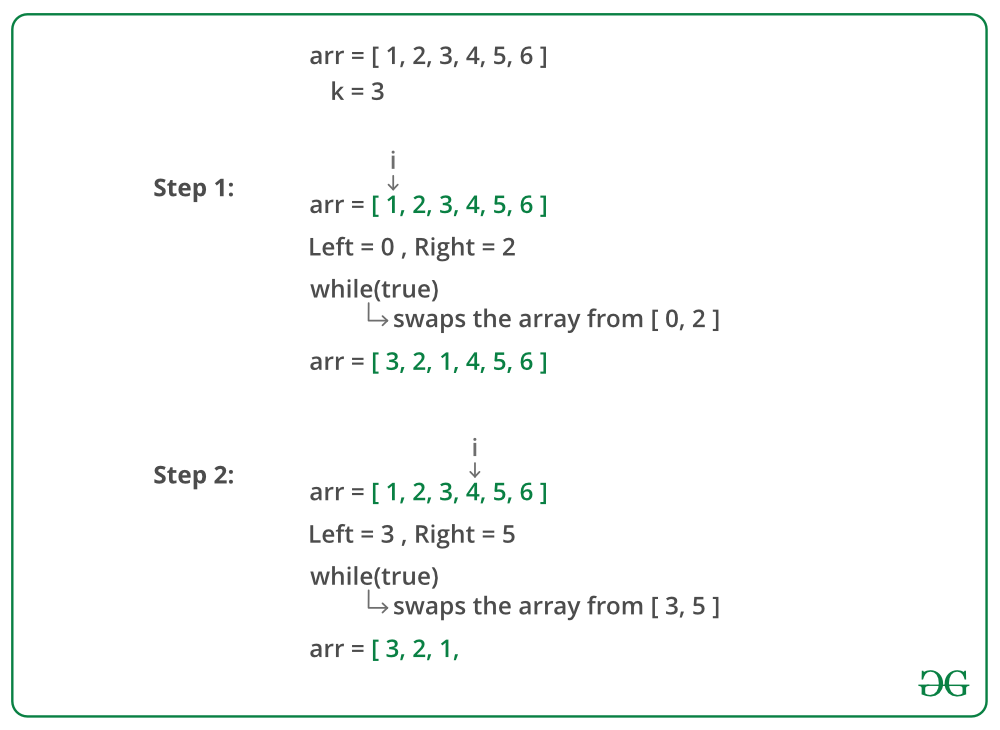
Reverse An Array In Groups Of Given Size Geeksforgeeks

How To Print Last Element In Set In C Stack Overflow
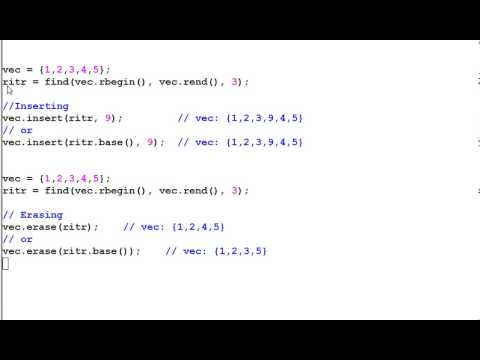
Learn Stl Tricky Reverse Iterator Youtube
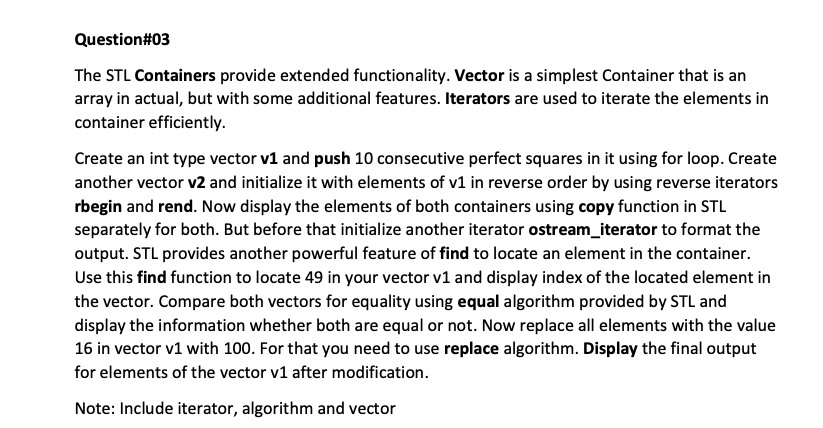
Solved Question 03 The Stl Containers Provide Extended Fu Chegg Com
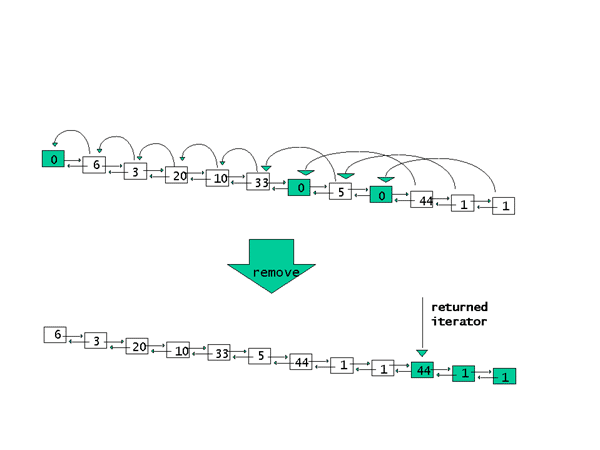
Angelikalanger Com Are Set Iterators Mutable Or Immutable Angelika Langer Training Consulting
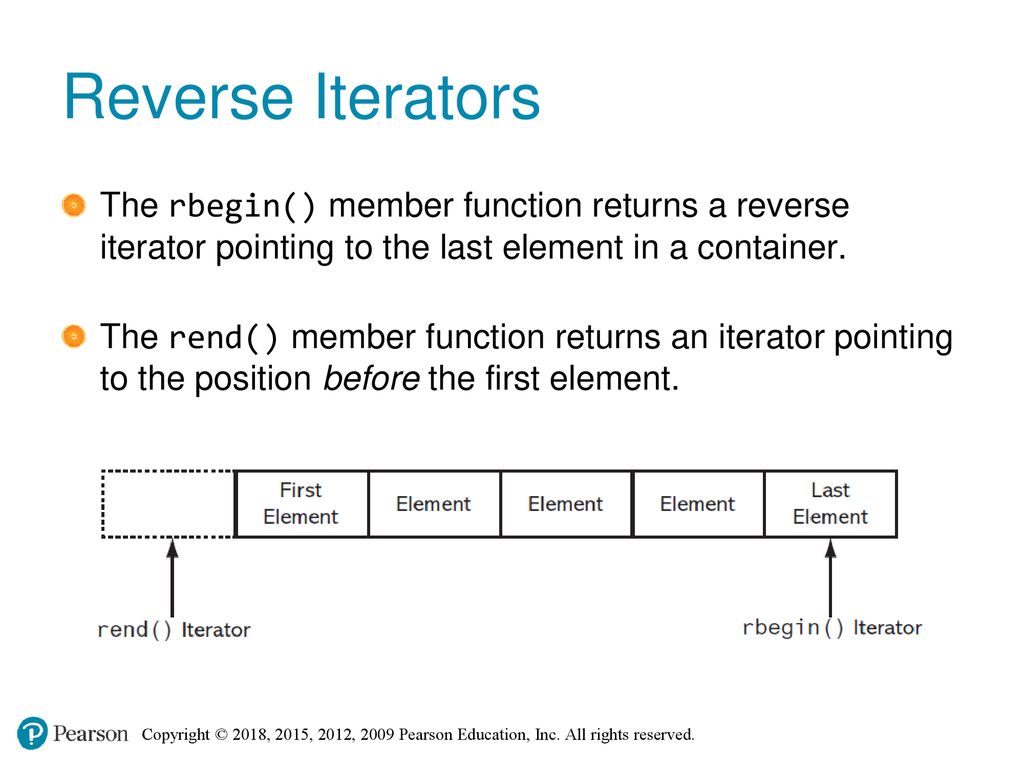
The Standard Template Library Ppt Download
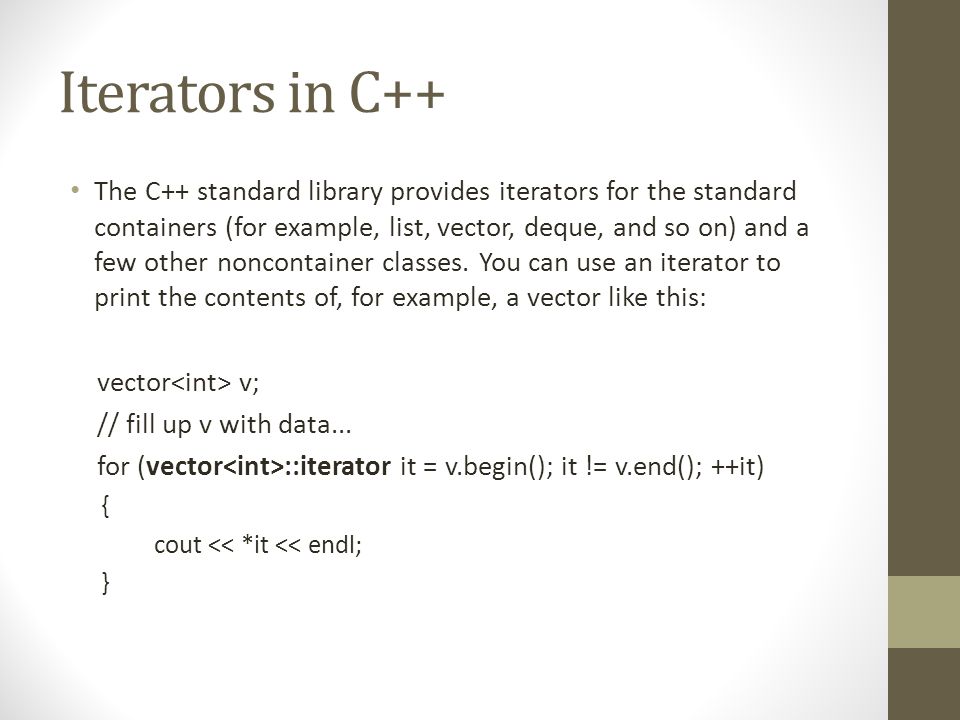
Iterators Iterator An Iterator In C Is A Concept That Refines The Iterator Design Pattern Into A Specific Set Of Behaviors That Work Well With The Ppt Download
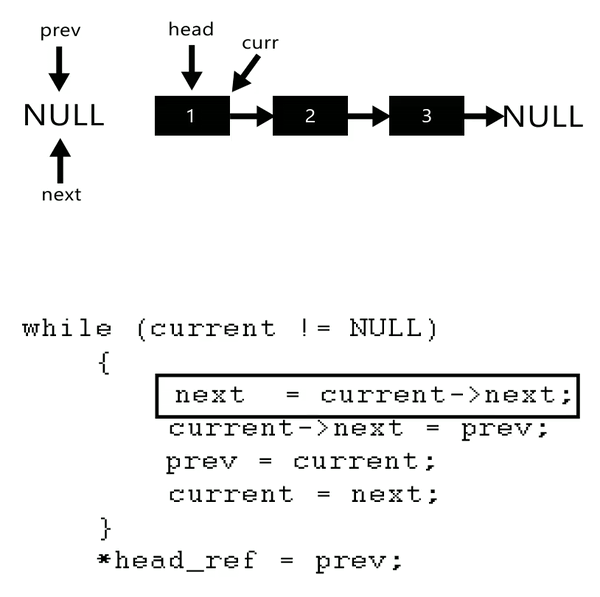
Q Tbn 3aand9gcshiekoa8t9tw9a13j Openqolwkpsbzmeyfw Usqp Cau

Libstdc V3 Source Templatestd Set Key Compare Alloc Class Reference
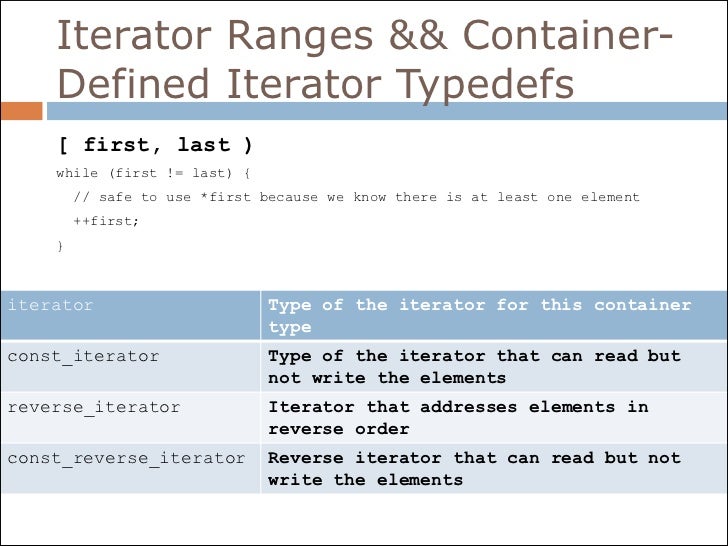
An Introduction To Part Of C Stl
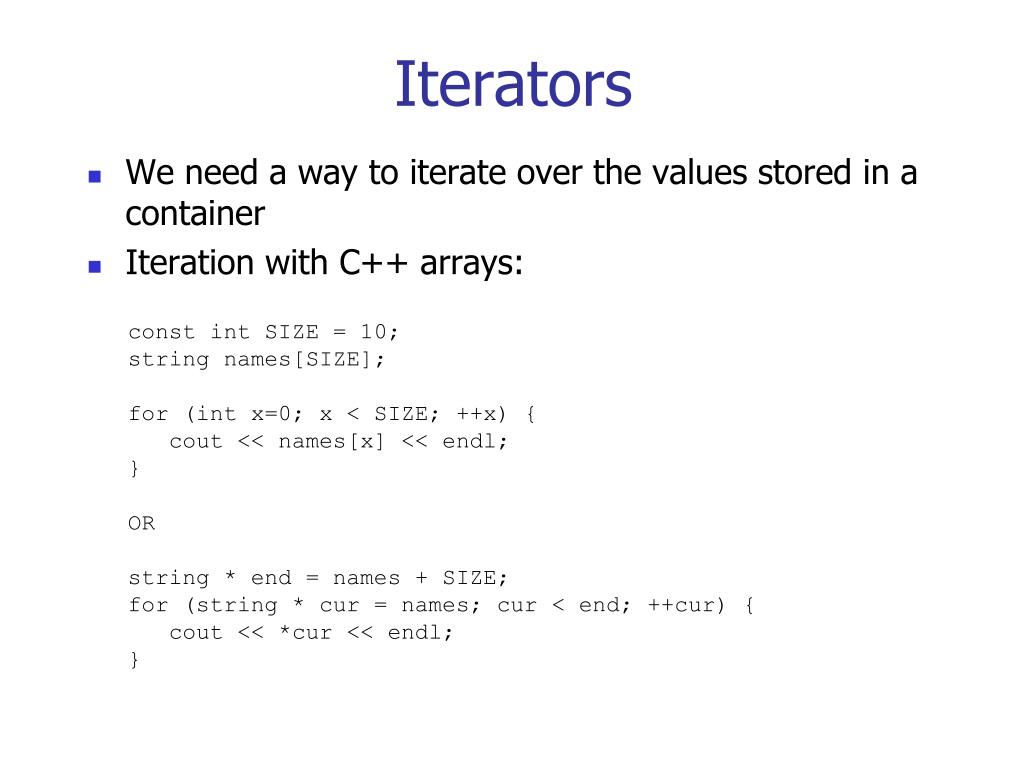
Ppt Standard Template Library Stl Powerpoint Presentation Free Download Id

The Foreach Loop In C Journaldev

Iterator Pattern Wikipedia
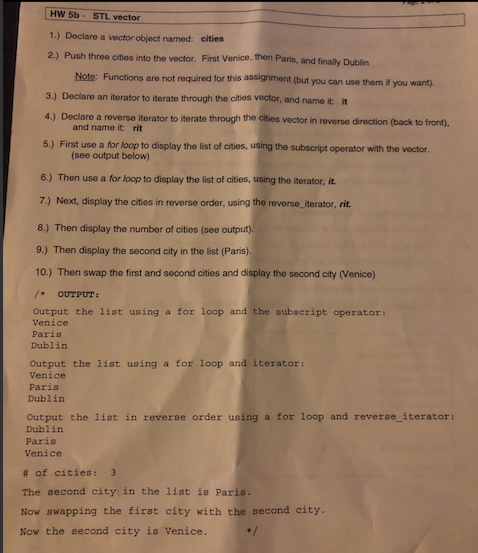
Solved Code Should Be In C Please Show A Screenshot Of Chegg Com
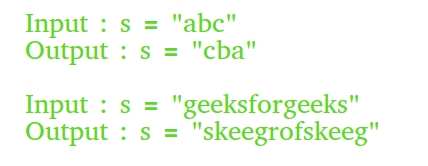
Different Methods To Reverse A String In C C Geeksforgeeks
C Iterator Programmer Sought
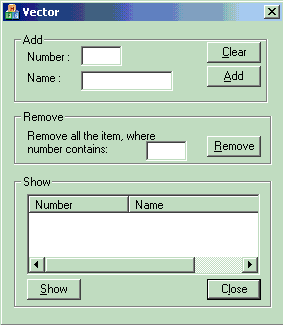
A Study Of Stl Container Iterator And Predicates Codeproject
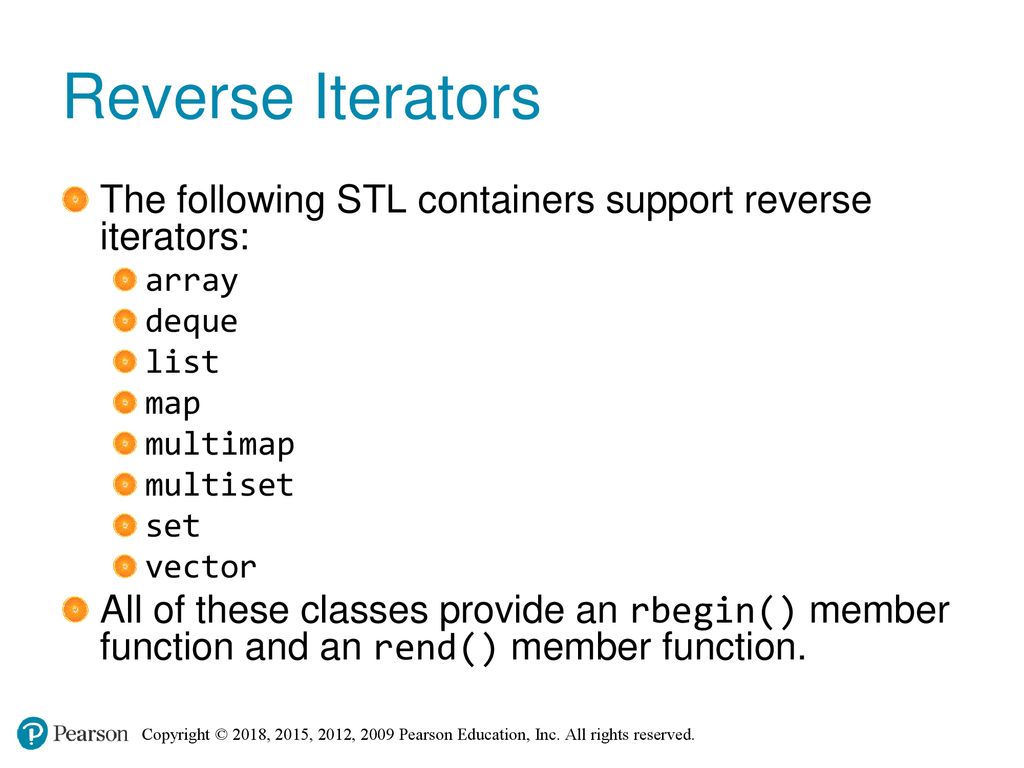
The Standard Template Library Ppt Download

Iterator Facade And Adaptor Boost
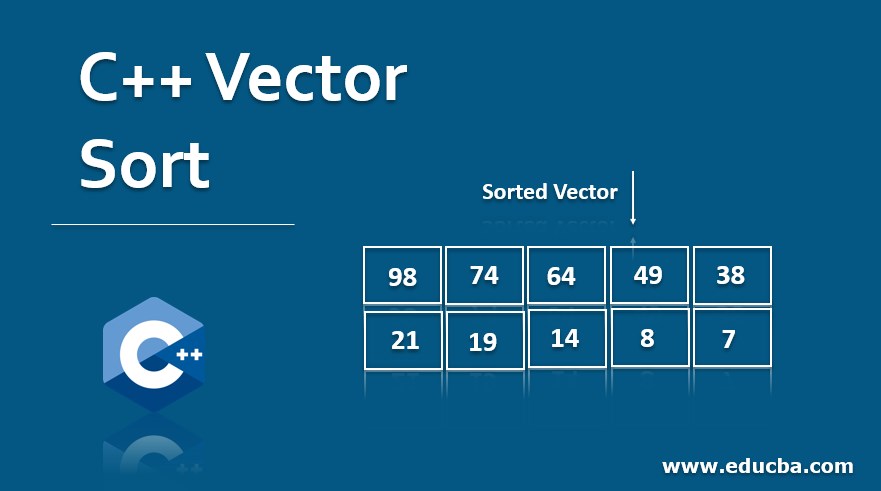
C Vector Sort How Does Vector Sorting Work In C Programming
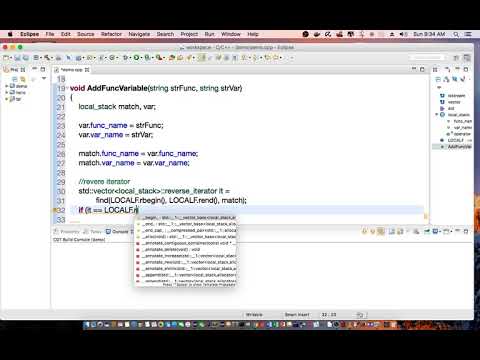
C Reverse Iterator Tutorial Youtube
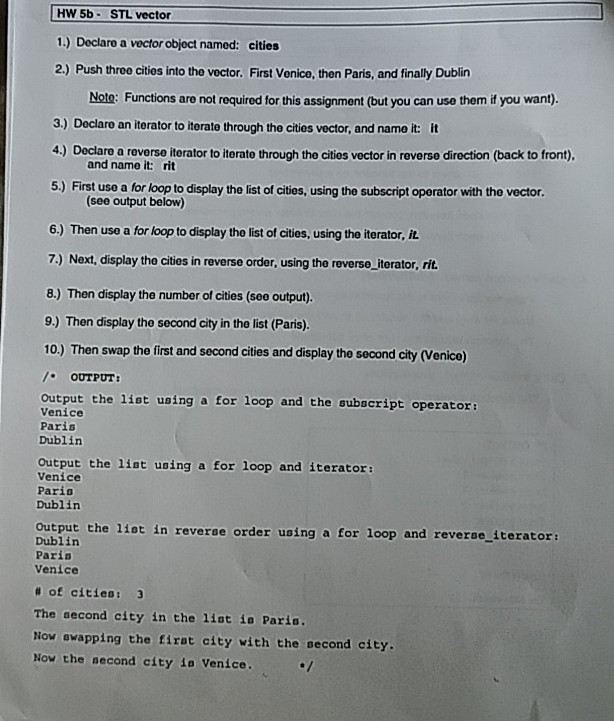
Solved Hw 5b Stl Vector 1 Declare A Vectorobjoct Named Chegg Com

Qmapiterator Class Qt 4 8
How To Traverse A C Set In Reverse Direction Geeksforgeeks
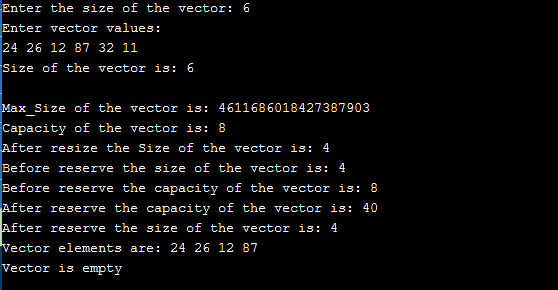
C Vector Example Vector In C Tutorial
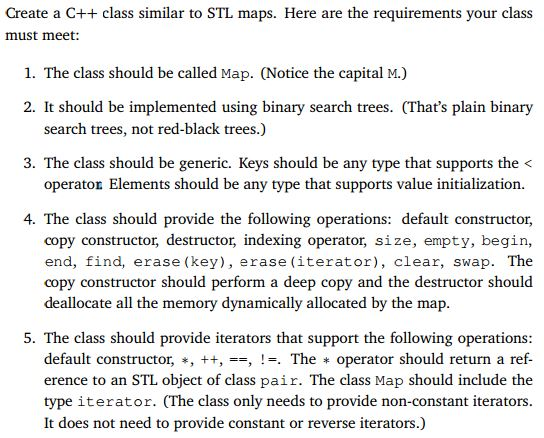
Solved Create A C Class Similar To Stl Maps Here Are T Chegg Com
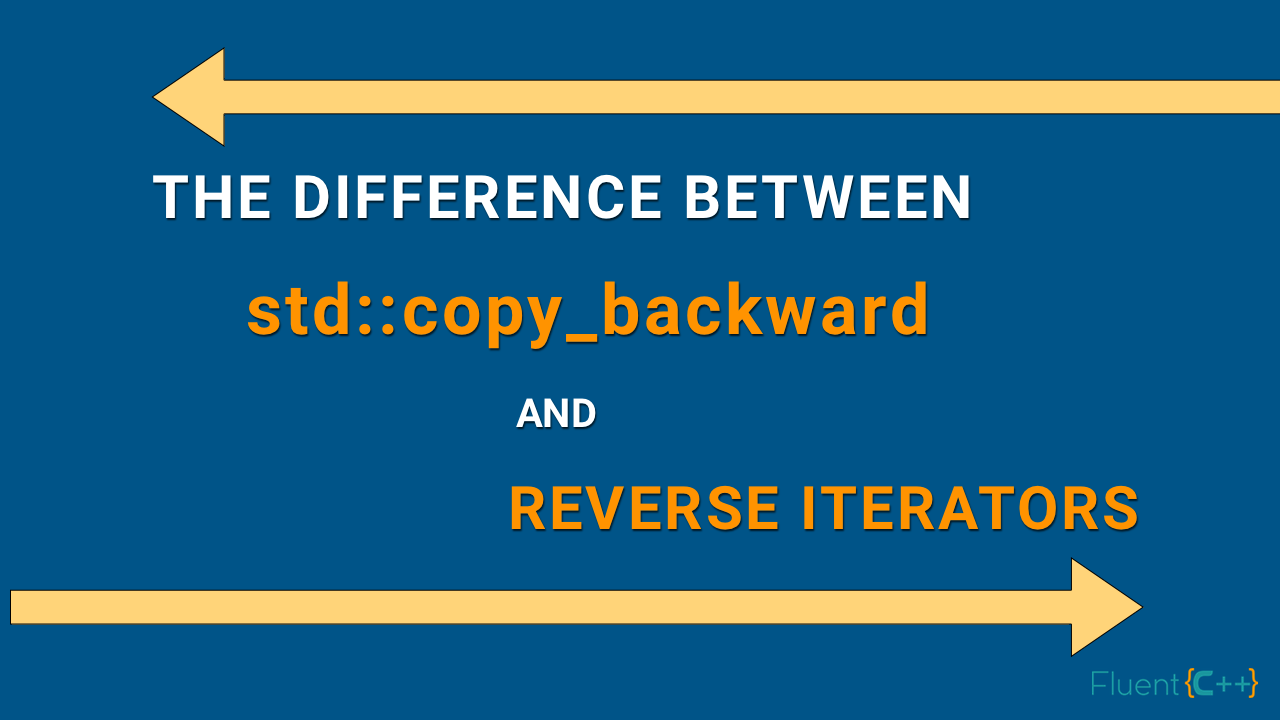
The Difference Between Std Copy Backward And Std Copy With Reverse Iterators Fluent C

Vectors In Stl
1
Ninova Itu Edu Tr Tr Dersler Bilgisayar Bilisim Fakultesi 336 Ise 103 Ekkaynaklar G6

Writing Standard Library Compliant Data Structures And Algorithms Ppt Download
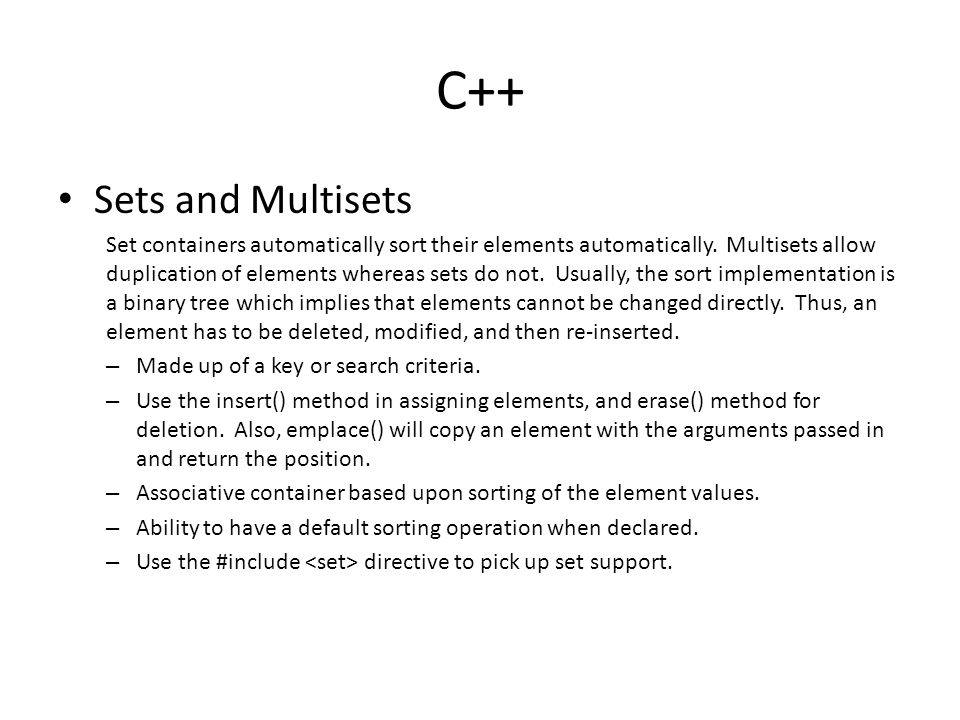
C Sets And Multisets Set Containers Automatically Sort Their Elements Automatically Multisets Allow Duplication Of Elements Whereas Sets Do Not Usually Ppt Download

Python Program To Iterate Over The List In Reverse Order Codevscolor
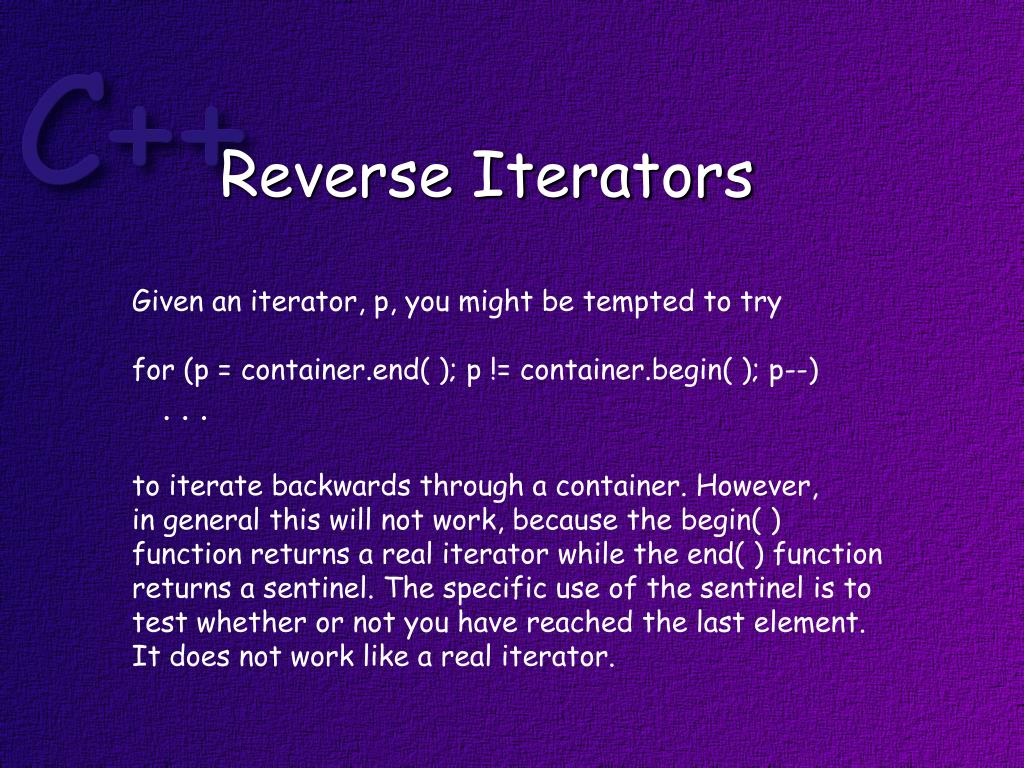
Ppt The Standard Template Library Powerpoint Presentation Free Download Id 949

Learn Stl Tricky Reverse Iterator Youtube

Set In C Stl The Complete Reference Journaldev
Http Www Cis Gvsu Edu Moorejar Public Cis263 Javatoc 11 Pdf
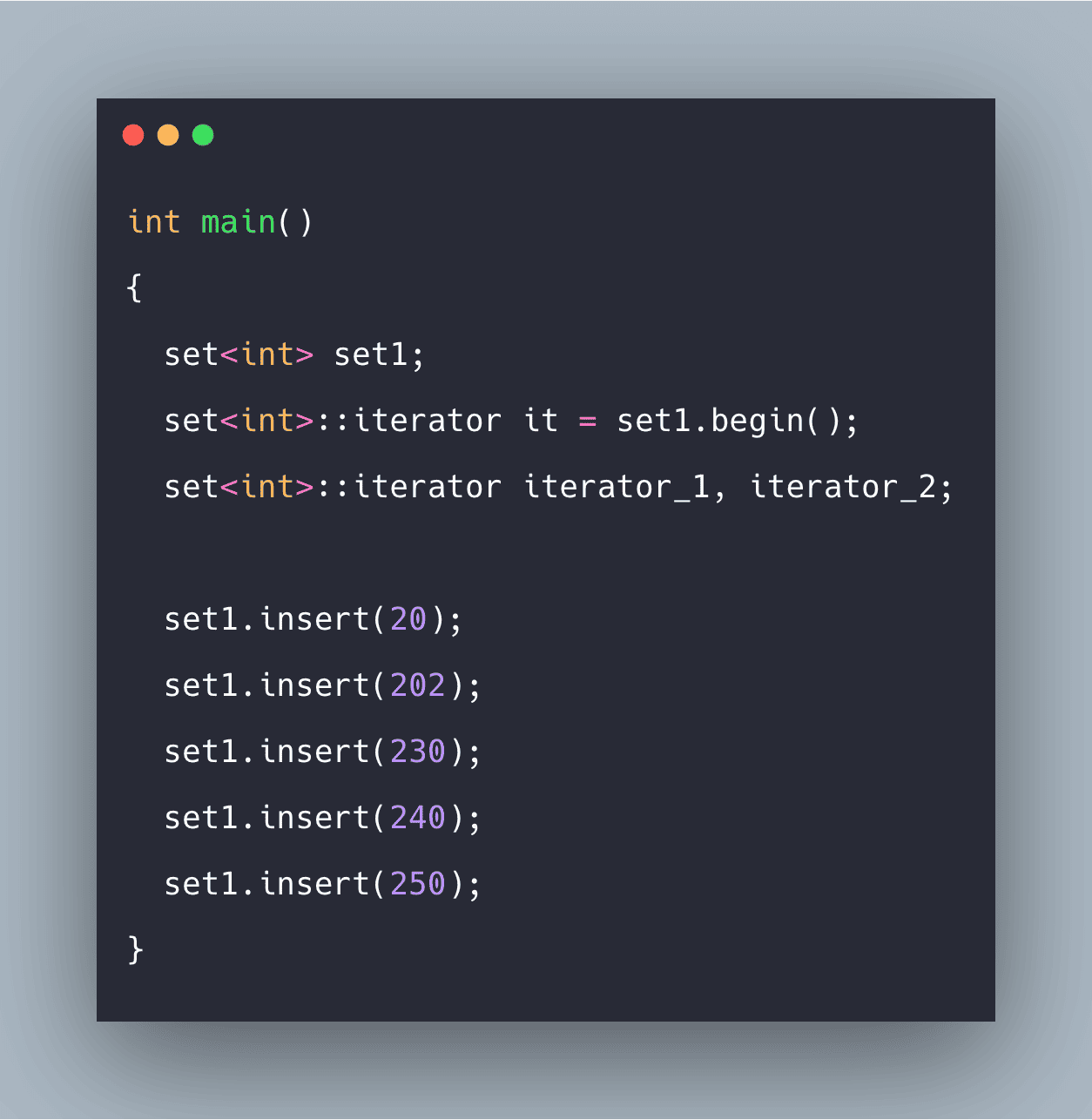
C Set Example Sets In C Program
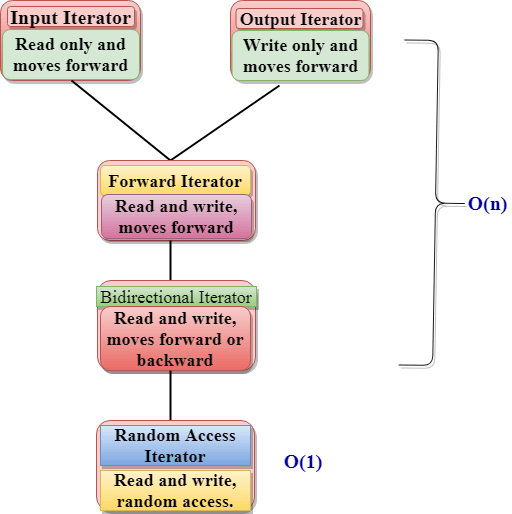
C Iterators Javatpoint
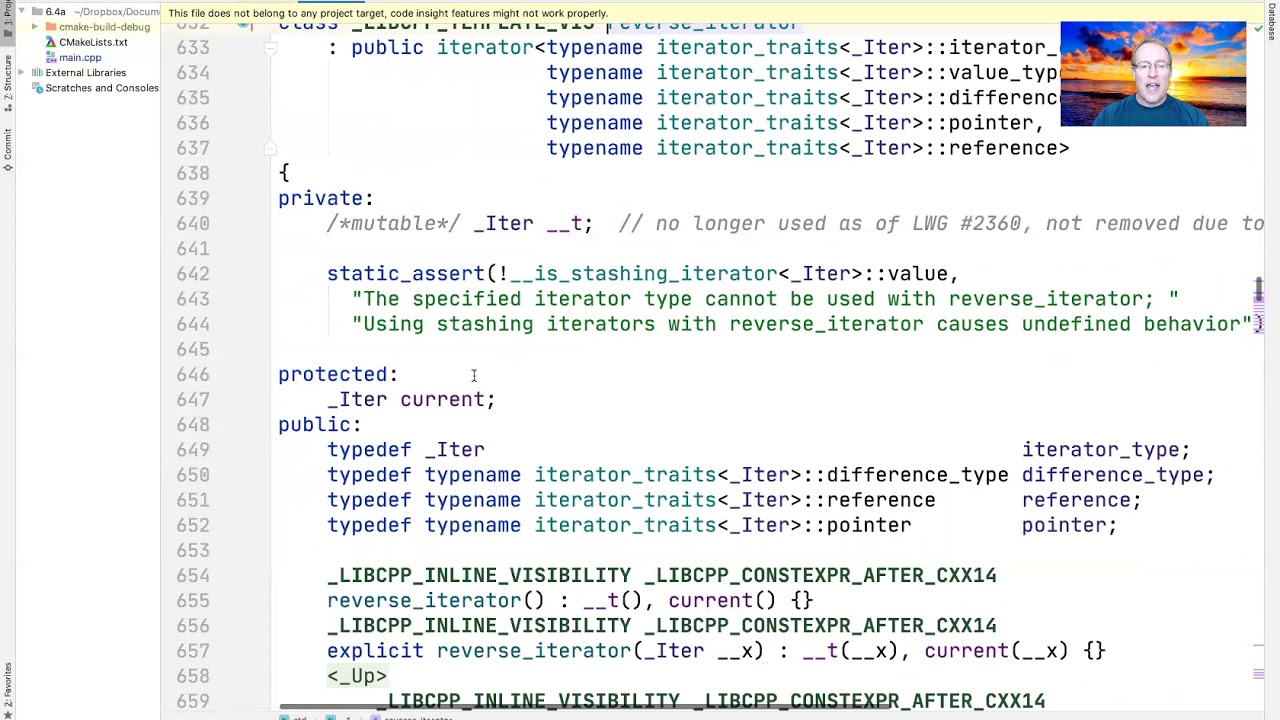
C Stl Iterator Adapters Part 2 Youtube
Q Tbn 3aand9gcs2duvw8aibqba4ipqzu U73qkfsysesg6v S0ilkbeccwf4a Usqp Cau
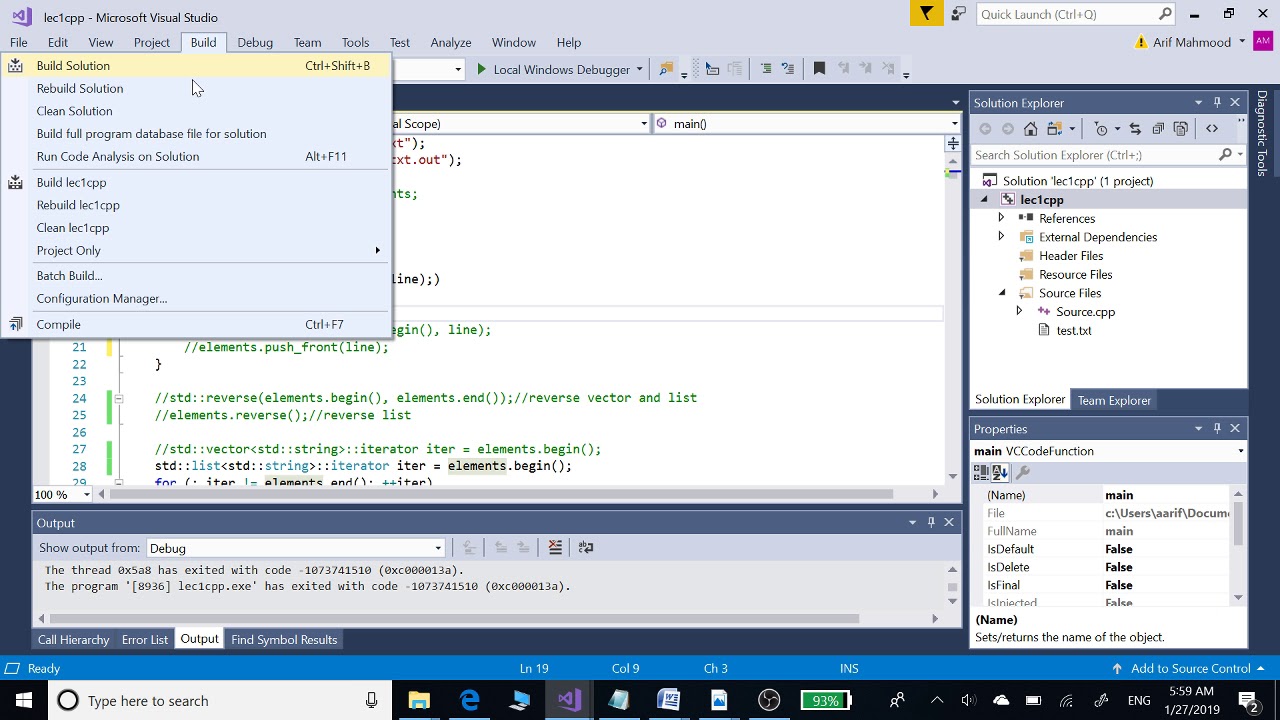
Reverse Vector List In C And C With Inserting At Front Of Vectors And Lists 58 Youtube
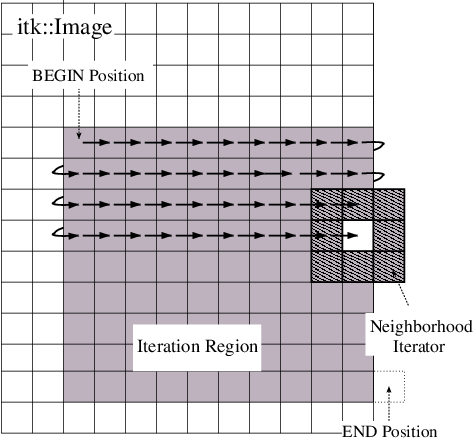
Iterators Orfeo Toolbox 7 2 0 Documentation
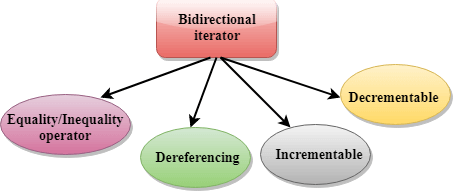
C Bidirectional Iterator Javatpoint
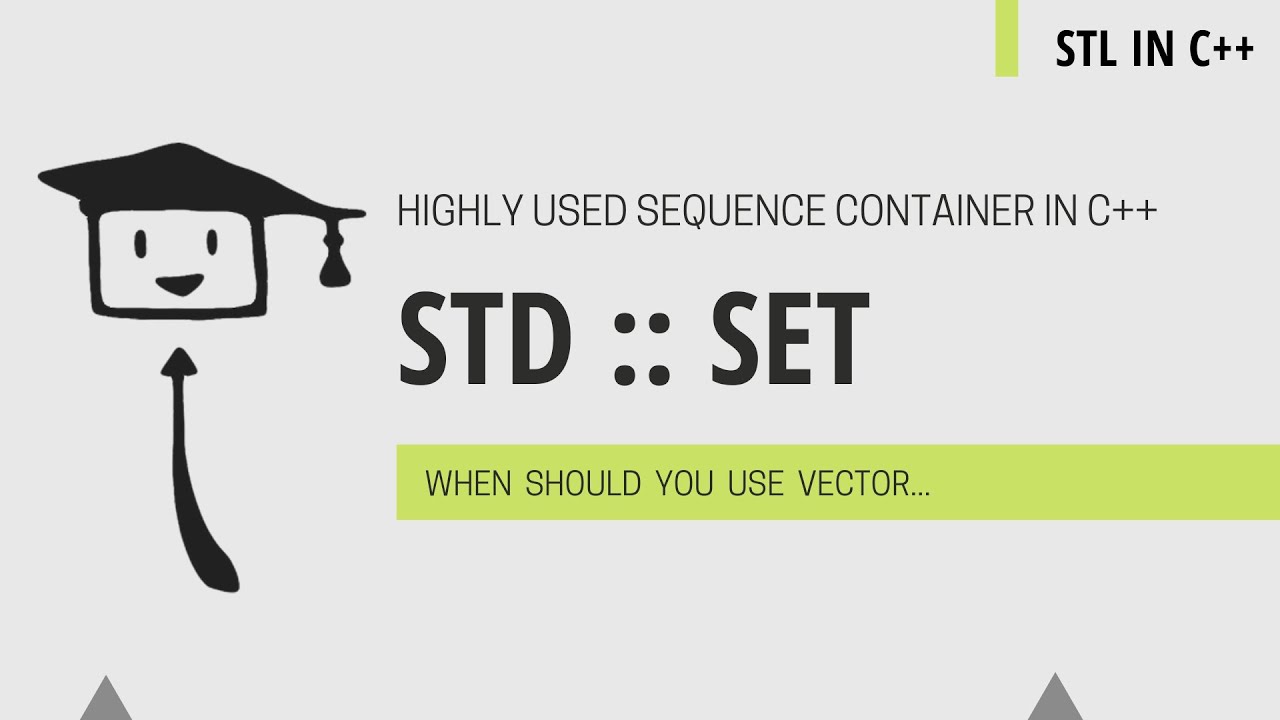
Std Set In C Stl C Youtube

C Std Array Declare Initialize Passing Std Array To Function

Standard Template Library Ppt Download
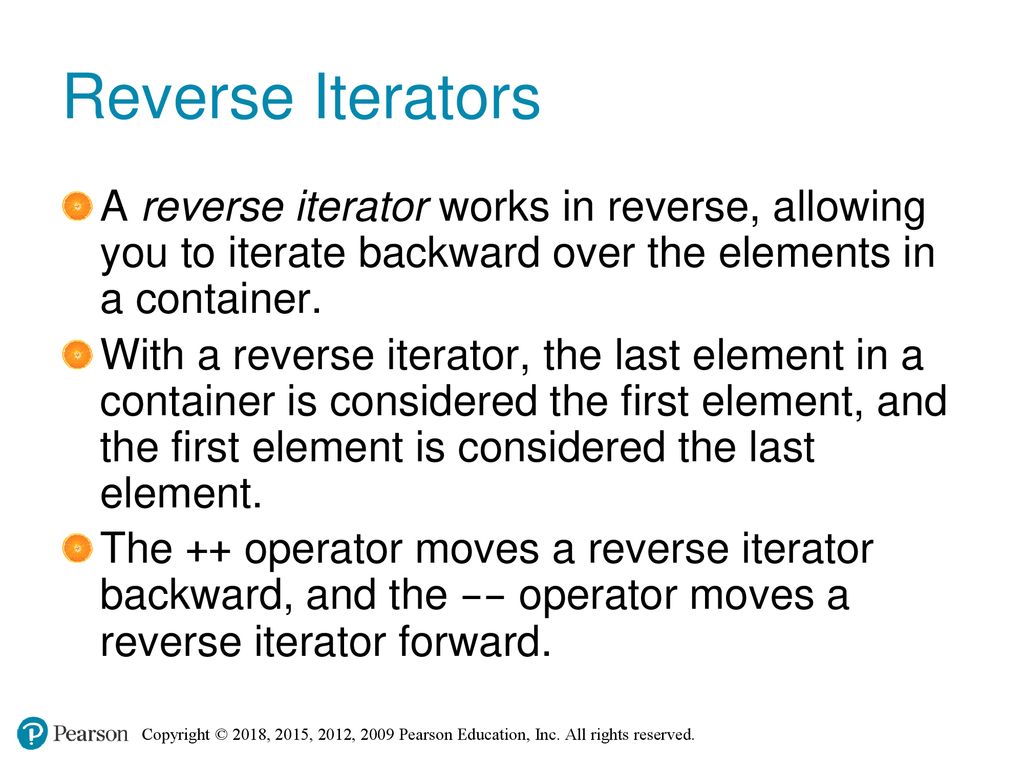
The Standard Template Library Ppt Download
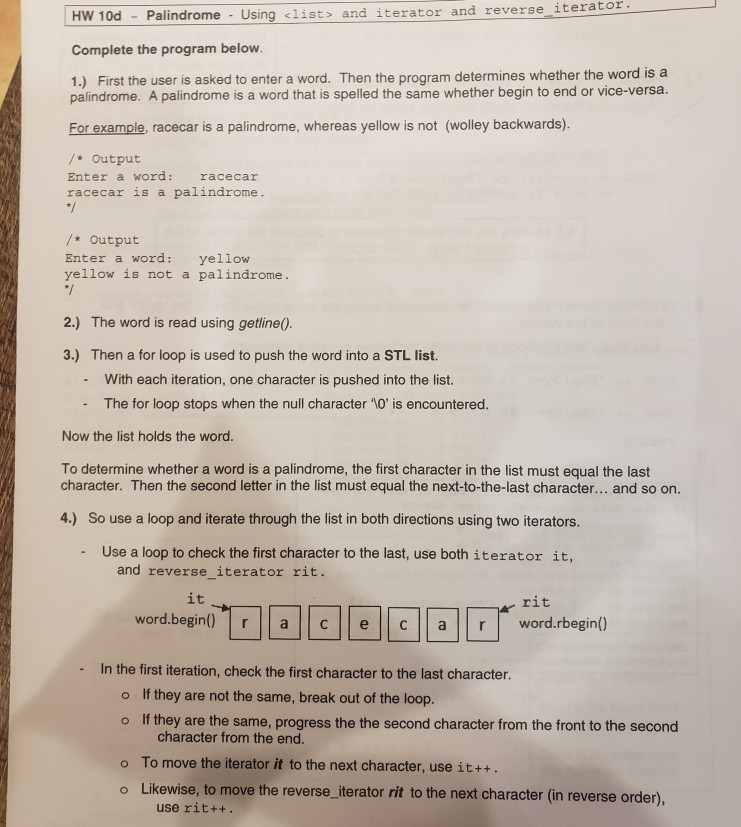
Solved Hw 10d Palindrome Using List And Iterator And Chegg Com
H Cpp Std Vector
C Stl Containers Summary Vector List Deque Queue Stack Set Map Programmer Sought
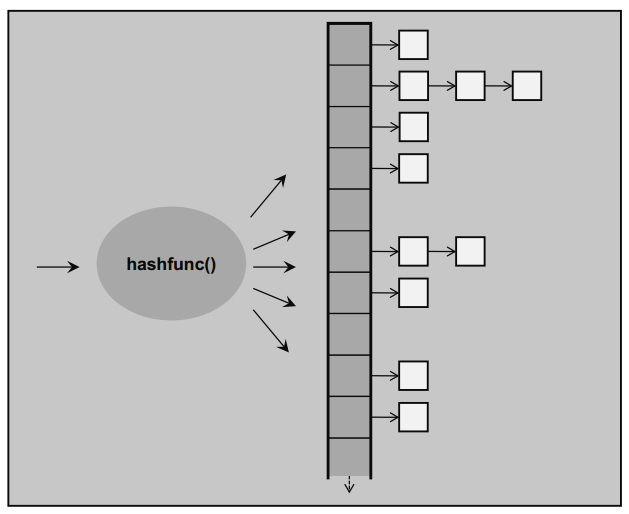
Stl Unordered Container
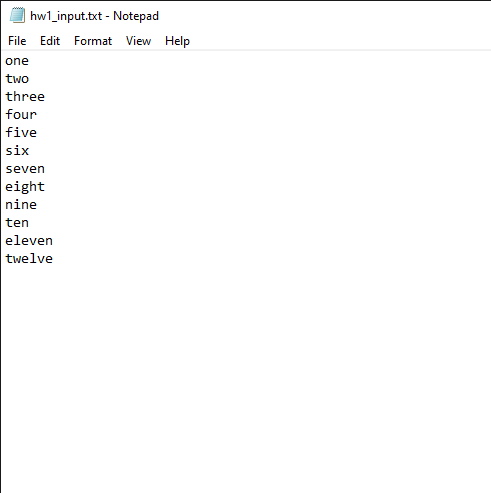
Solved C Add The Following Class Vector In The Vector H Chegg Com

Inherit All Functions From User Defined Iterator In Reverse Iterator Stack Overflow

Introduction To C C Lecture 6 Stl Jjcao Ppt Download
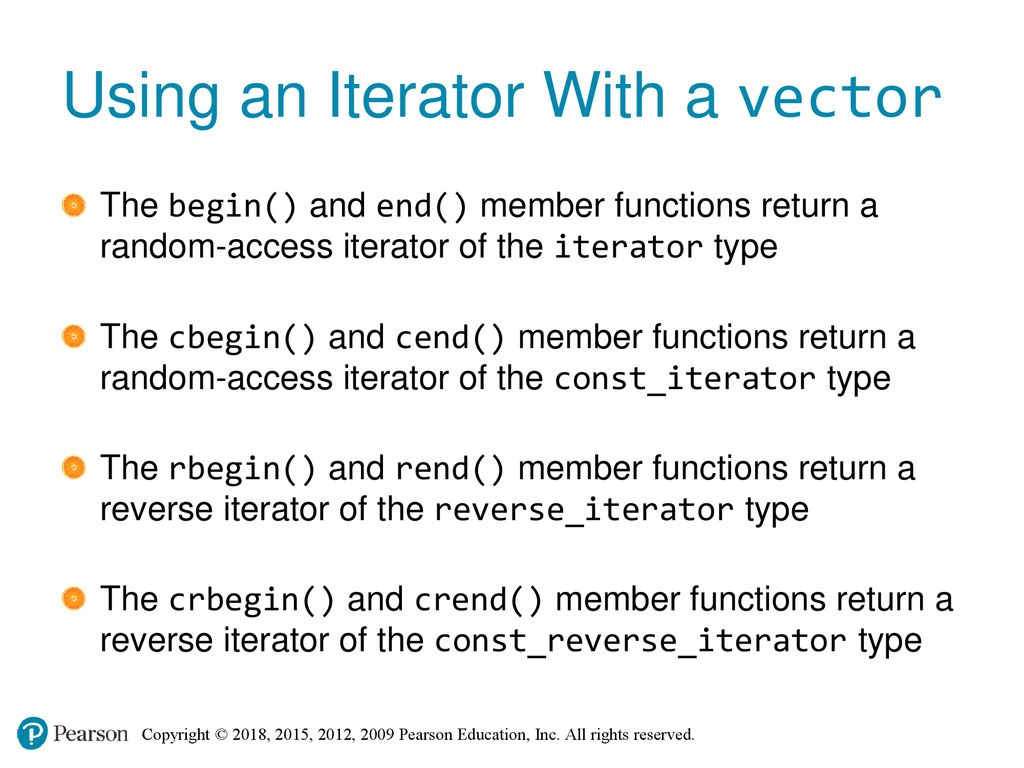
The Standard Template Library Ppt Download
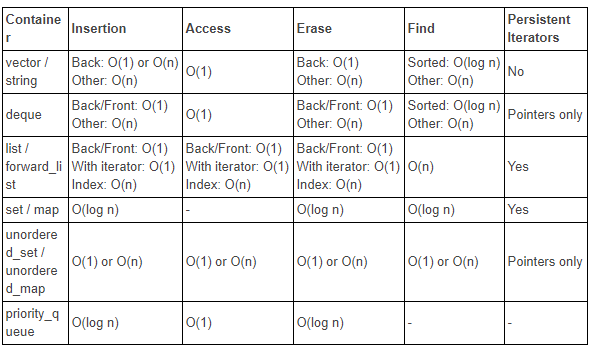
Stl Container Performance Stl Container Performance Table By Onur Uzun Medium
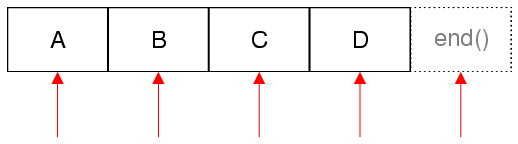
Container Classes Qt Core 5 15 1

Writing Standard Library Compliant Data Structures And Algorithms Ppt Download
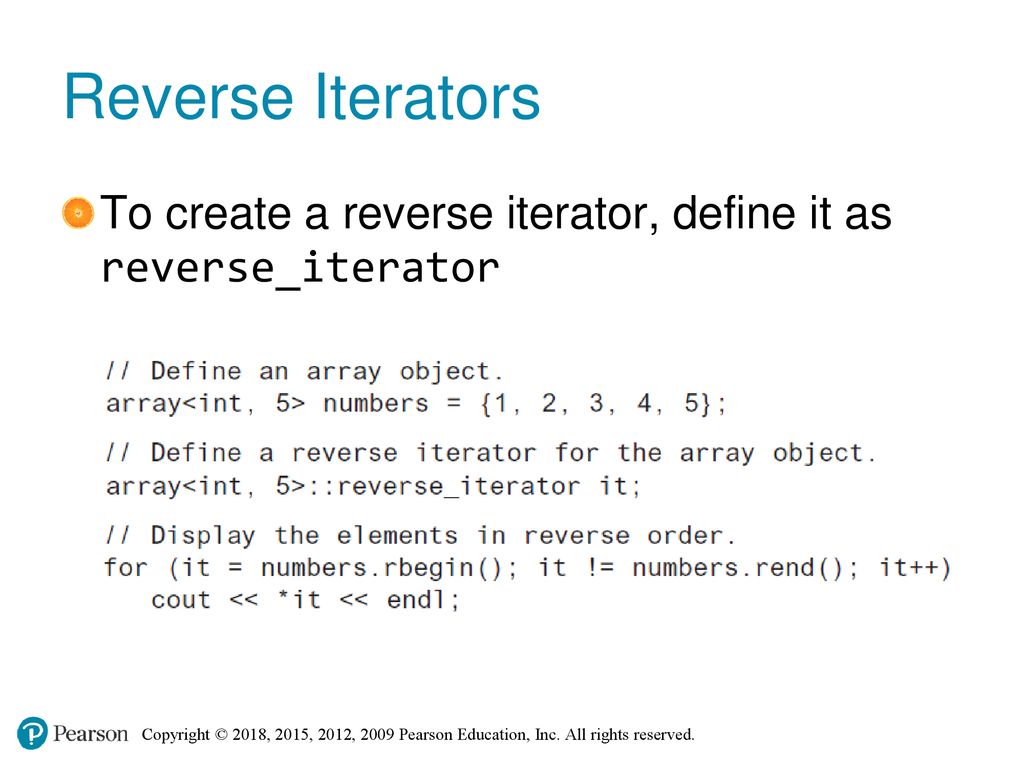
The Standard Template Library Ppt Download
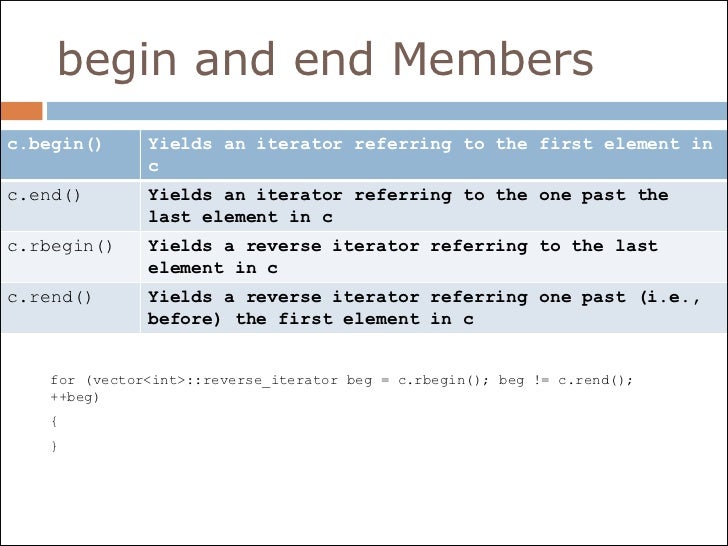
An Introduction To Part Of C Stl
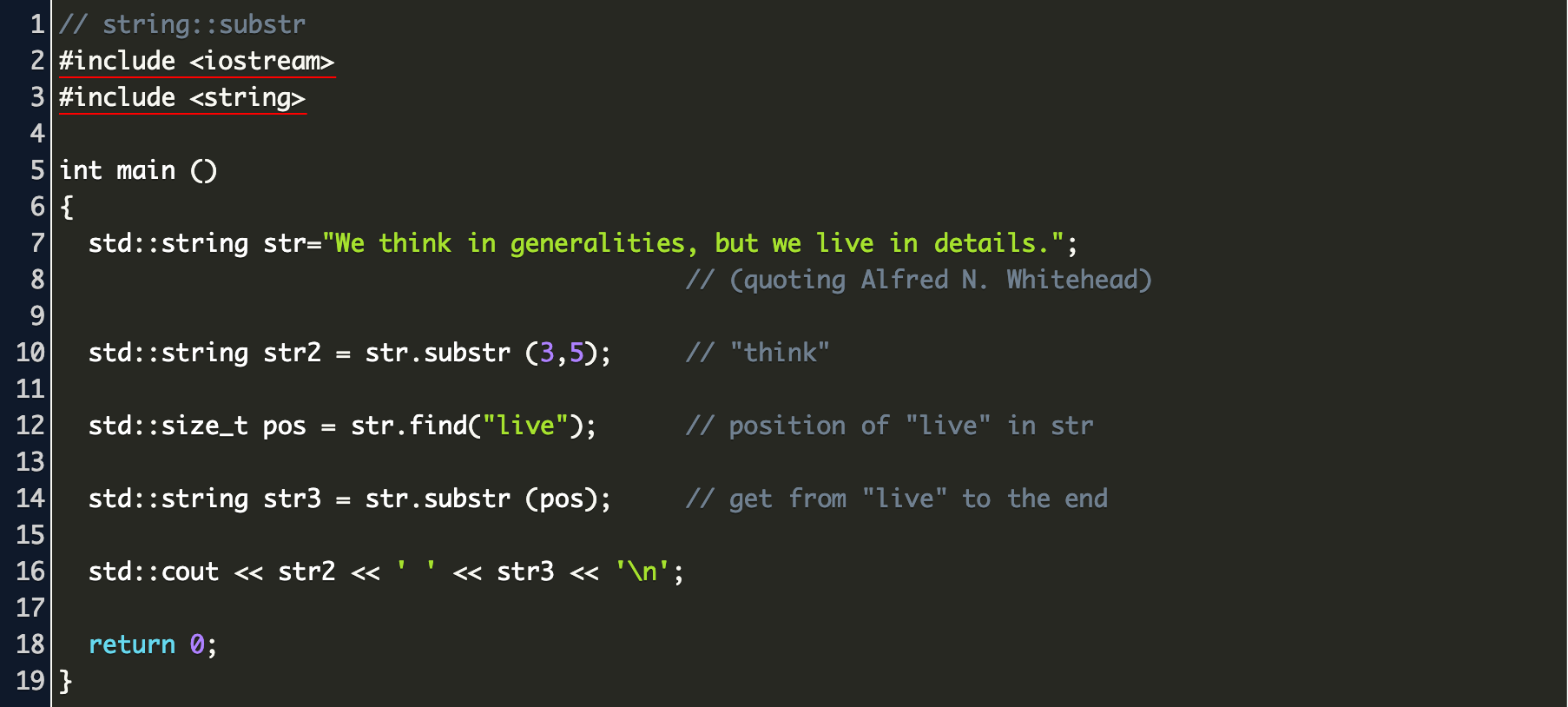
Create Copy Of Range Of String C Code Example

C Program To Reverse A String
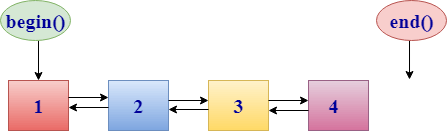
C Iterators Javatpoint
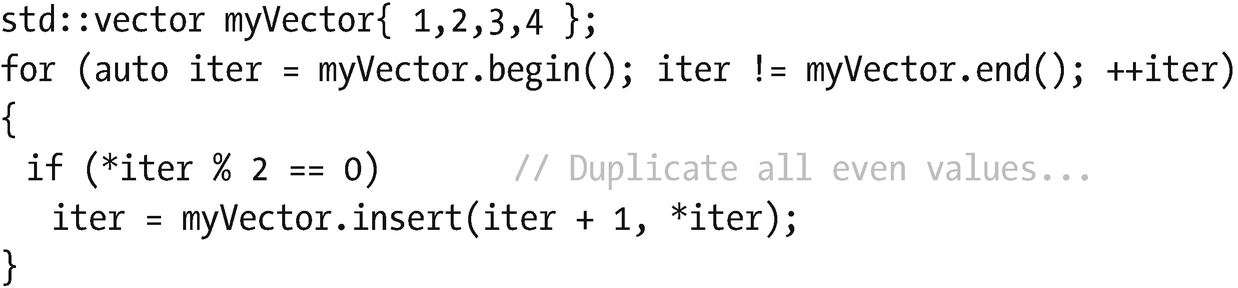
Containers Springerlink
Http Www Aristeia Com Papers Cuj June 01 Pdf

How To Call Erase With A Reverse Iterator Stack Overflow
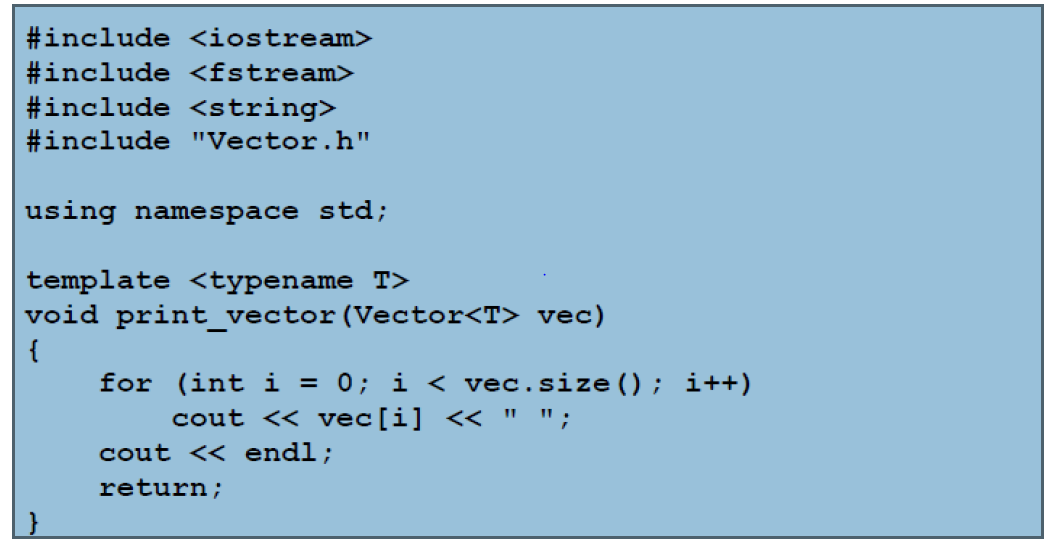
Solved Please Use C Please Help Thank You So Much Ad Chegg Com